Testing ChatGPT 4.5, Simulating Colorful Bouncing Balls in p5.js
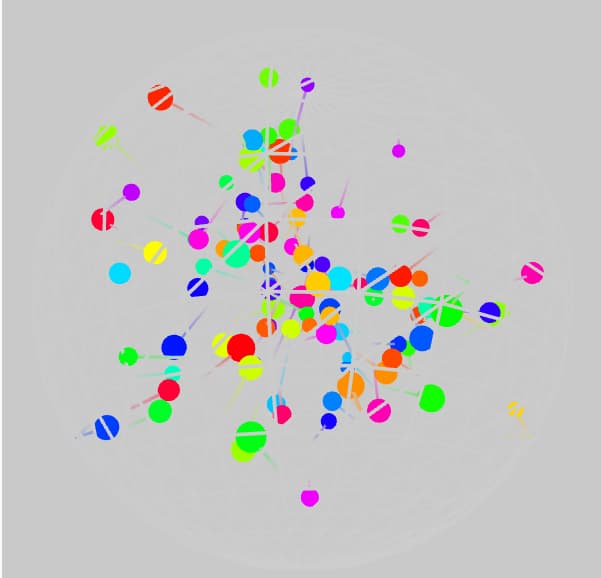
The Prompt: A Technical p5.js Task
I provided ChatGPT 4.5 with the following prompt:
“Write a p5.js script that simulates 100 colorful balls bouncing inside a sphere. Each ball should leave behind a fading trail showing its recent path. The container sphere should rotate slowly. Make sure to implement proper collision detection so the balls stay within the sphere.”
This required a blend of 3D graphics, physics-based simulation, and visual design, making it a robust test of the AI’s coding abilities in p5.js.
ChatGPT 4.5’s Response: A Detailed p5.js Script
ChatGPT 4.5 delivered a comprehensive p5.js script with clear comments and an explanatory note. The code is structured as follows:
let balls = [];
const numBalls = 100;
const sphereRadius = 200;
let angleX = 0;
let angleY = 0;
function setup() {
createCanvas(600, 600, WEBGL);
colorMode(HSB, 360, 100, 100, 100);
for (let i = 0; i < numBalls; i++) {
balls.push(new Ball());
}
}
function draw() {
background(0, 0, 0, 20); // transparent background for fading trail
orbitControl(); // allow mouse interaction
// slow auto rotation
angleX += 0.002;
angleY += 0.003;
rotateX(angleX);
rotateY(angleY);
noFill();
stroke(0, 0, 80, 50);
sphere(sphereRadius); // draw container sphere
for (let ball of balls) {
ball.update();
ball.checkBoundary();
ball.display();
}
}
class Ball {
constructor() {
this.pos = p5.Vector.random3D().mult(random(sphereRadius * 0.8));
this.vel = p5.Vector.random3D().mult(random(1, 3));
this.size = random(5, 10);
this.col = color(random(360), 100, 100);
this.history = [];
this.maxHistory = 20;
}
update() {
this.pos.add(this.vel);
// add position to history
this.history.push(this.pos.copy());
if (this.history.length > this.maxHistory) {
this.history.shift();
}
}
checkBoundary() {
let distFromCenter = this.pos.mag();
if (distFromCenter + this.size / 2 > sphereRadius) {
// correct the position to stay within the boundary
let normal = this.pos.copy().normalize();
let velocityAlongNormal = this.vel.dot(normal);
this.vel.sub(p5.Vector.mult(normal, 2 * velocityAlongNormal)); // reflect velocity vector
this.pos = normal.mult(sphereRadius – this.size / 2 – 1); // reposition slightly inside sphere
}
}
display() {
// display fading trail
beginShape();
noFill();
stroke(this.col);
strokeWeight(2);
for (let i = 0; i < this.history.length; i++) {
let pos = this.history[i];
let alpha = map(i, 0, this.history.length, 0, 100);
stroke(hue(this.col), saturation(this.col), brightness(this.col), alpha * 0.5);
vertex(pos.x, pos.y, pos.z);
}
endShape();
// display ball
push();
translate(this.pos.x, this.pos.y, this.pos.z);
noStroke();
fill(this.col);
sphere(this.size);
pop();
}
}
ChatGPT 4.5 provided a concise breakdown of the script’s functionality:
- It initializes 100 balls with random positions, velocities, sizes, and colors within a spherical container.
- Each ball maintains a history of its recent positions (up to 20 points) to create fading trails.
- Collision detection ensures balls remain within the sphere by reflecting their velocities upon boundary contact.
- The sphere rotates slowly in 3D, visualized using WebGL, with transparency for the trails.
- The script’s structure and comments facilitate understanding and potential modifications.
The Cost: A Barrier to Recommendation
ChatGPT 4.5’s API pricing is notably high, at $75 per million input tokens and $150 per million output tokens. For my specific prompt and response, the cost was only $0.00638, which appears low due to the minimal token usage. However, the per-token rates are prohibitively expensive for broader or frequent coding tasks. Given this cost structure, I cannot recommend ChatGPT 4.5 for general coding purposes, as it would quickly become cost-prohibitive for developers, particularly those working on multiple projects or larger-scale applications.
Testing OpenAI’s ChatGPT 4.5 with this p5.js prompt revealed its strong technical capabilities, producing a functional and visually representative script for simulating colorful bouncing balls. The static image corroborates the code’s ability to generate a colorful, trail-laden pattern within a spherical boundary, as described. However, the API’s high cost—$75 per million input tokens and $150 per million output tokens—makes it impractical for widespread coding use, even with the low cost of $0.00638 for this specific interaction. While ChatGPT 4.5 excels in precision and clarity, its expense prevents me from recommending it for coding projects, particularly for developers on a budget or working at scale. For specialized, one-off tasks with careful cost management, it remains a powerful tool, but broader adoption is limited by its pricing structure.